I have been attempting to do the Micro Blog exercise in the java syllabus for about 2 days now and I honestly have no idea where to go from where I am at. This is the closest I think I have gotten to a solution but am not quite sure why I am failing the tests. This is my code:
import java.util.stream.IntStream;
class MicroBlog {
public String truncate(String input) {
IntStream stream = input.codePoints().limit(5);
StringBuilder strBuilder = new StringBuilder();
strBuilder.append(stream);
return strBuilder.toString();
}
}
And this is the message I get when failing the first test:
Message: expected:<[brĂĽh]e> but was:<[java.util.stream.SliceOps$2@12843fc]e>
Exception: org.junit.ComparisonFailure: expected:<[brĂĽh]e> but was:<[java.util.stream.SliceOps$2@12843fc]e>
at MicroBlogTest.germanLanguageShort_broth(MicroBlogTest.java:27)
Welcome to the forum!
When you submit an exercise via the Exercism CLI you can request mentoring on a solution even if it fails the tests. Can help you get unstuck on situations like this. I don’t mind offering help here on the forum to get you unstuck, but keep in mind that you probably want mentors of the Java track to take a look at it. I usually mentor the Java track so I can provide a bit of help here.
Your solution is more than halfway there. You have the right idea of making a stream with the codepoints and then using a string builder to build the final string. However, the problem with your solution lies in this line:
strBuilder.append(stream);
stream
is an IntStream
, and this does beg the question “does StringBuilder know how to append IntStreams”? If you look at the docs for StringBuilder, you see a lot of definitions for append
, but none takes an IntStream
. This is the first clue that StringBuilder
might not know how to append IntStream
s. But your code compiles, so it’s clearly using some of those definitions of append
. The definition it is using is this one, because it is the one that fits:
append(Object obj)
In case of doubt, your IDE can also help you figure out which definition of the function it is using:
VSCode:

Intellij:
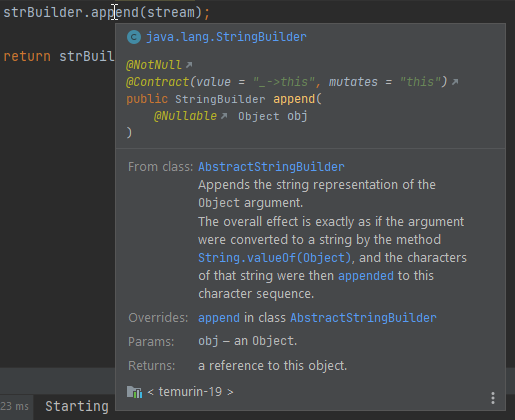
Reading the description for this definition of append
:
Appends the string representation of the Object argument.
So, basically, this definition of append
is seeing the IntStream
as just an Object
and appending its string representation of this object to the string builder. In the error message you see this representation of the object: java.util.stream.SliceOps$2@12843fc
, which of course is not what you want. You want a string representation that actually sees the ints in the IntStream
as characters and create a string with it.
However, note that StringBuilder
actually has a method that can help us here: StringBuilder.appendCodePoint(int) which appends a single int
representing a codepoint to the string builder! All you have to do is to find a way to extract all the ints from the stream and pass each one to appendCodePoint
. There are several ways to do this and I’ll let you have a go at figuring how this could work.
1 Like